1.23 Write a program that asks the user to enter two numbers, obtains the two numbers from the user and prints the sum, product,
difference, and quotient of the two numbers.
Exercise 1.23 Solution
#include <iostream>
using std::cout;
using std::endl;
using std::cin;
int main()
{
int num1, num2; // declare variables
cout << "Enter two integers: "; // prompt user
cin >> num1 >> num2; // read values from keyboard
// output the results
cout << "The sum is " << num1 + num2
<< "\nThe product is " << num1 * num2
<< "\nThe difference is " << num1 - num2
<< "\nThe quotient is " << num1 / num2 << endl;
return 0; // indicate successful termination
}
Enter two integers: 8 22
The sum is 30
The product is 176
The difference is -14
The quotient is 0
1.24 Write a program that prints the numbers 1 to 4 on the same line with each pair of adjacent numbers separated by one space.
Write the program using the following methods:
a) Using one output statement with one stream insertion operator.
b) Using one output statement with four stream insertion operators.
c) Using four output statements.
//Exercise 1.24 Solution
#include <iostream>
using std::cout;
using std::endl;
int main ()
{
// Part A
cout << "1 2 3 4\n";
// Part B
cout << "1 " << "2 " << "3 " << "4\n";
// Part C
cout << "1 ";
cout << "2 ";
cout << "3 ";
cout << "4" << endl;
return 0;
}
1 2 3 4
1 2 3 4
1 2 3 4
1.25 Write a program that asks the user to enter two integers, obtains the numbers from the user, then prints the larger number
followed by the words “is larger.” If the numbers are equal, print the message “These numbers are equal.”
// Exercise 1.25 Solution
#include <iostream>
using std::cout;
using std::endl;
using std::cin;
int main()
{
int num1, num2; // declaration
cout << "Enter two integers: "; // prompt
cin >> num1 >> num2; // input to numbers
if ( num1 == num2 )
cout << "These numbers are equal." << endl;
if ( num1 > num2 )
cout << num1 << " is larger." << endl;
if ( num2 > num1 )
cout << num2 << " is larger." << endl;
return 0;
}
Enter two integers: 22 8
22 is larger.
1.26 Write a program that inputs three integers from the keyboard and prints the sum, average, product, smallest and largest of
these numbers. The screen dialogue should appear as follows:
Input three different integers: 13 27 14
Sum is 54
Average is 18
Product is 4914
Smallest is 13
Largest is 27
// Exercise 1.26 Solution
#include <iostream>
using std::cout;
using std::endl;
using std::cin;
int main()
{
int num1, num2, num3, smallest, largest; // declaration
cout << "Input three different integers: "; // prompt
cin >> num1 >> num2 >> num3; // input
largest = num1; // assume first number is largest
if ( num2 > largest ) // is num2 larger?
largest = num2;
if ( num3 > largest ) // is num3 larger?
largest = num3;
smallest = num1; // assume first number is smallest
if ( num2 < smallest )
smallest = num2;
if ( num3 < smallest )
smallest = num3;
cout << "Sum is " << num1 + num2 + num3
<< "\nAverage is " << (num1 + num2 + num3) / 3
<< "\nProduct is " << num1 * num2 * num3
<< "\nSmallest is " << smallest
<< "\nLargest is " << largest << endl;
return 0;
}
Input three different integers: 13 27 14
Sum is 54
Average is 18
Product is 4914
Smallest is 13
Largest is 27
1.27 Write a program that reads in the radius of a circle and prints the circle’s diameter, circumference and area. Use the constant
value 3.14159 for p. Do these calculations in output statements. (Note: In this chapter, we have discussed only integer constants
and variables. In Chapter 3 we will discuss floating-point numbers, i.e., values that can have decimal points.)
// Exercise 1.27 Solution
#include <iostream>
using std::cout;
using std::endl;
using std::cin;
int main()
{
int radius; // declaration
cout << "Enter the circle radius: "; // prompt
cin >> radius; // input
cout << "Diameter is " << radius * 2.0
<< "\nCircumference is " << 2 * 3.14159 * radius
<< "\nArea is " << 3.14159 * radius * radius << endl;
return 0;
}
Enter the circle radius: 8
Diameter is 16
Circumference is 50.2654
Area is 201.062
1.28 Write a program that prints a box, an oval, an arrow and a diamond as follows:
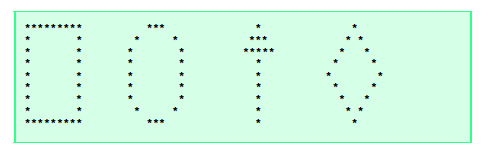
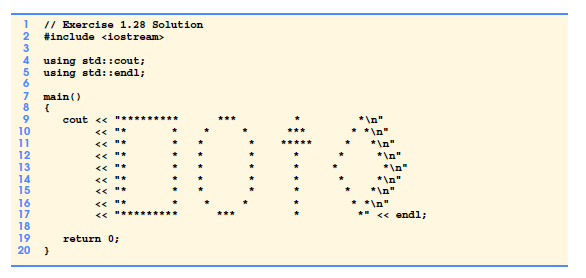
1.29 What does the following code print?
cout << “\n\n\n\n***\n”;
*
**
***
****
*****
1.30 Write a program that reads in five integers and determines and prints the largest and the smallest integers in the group. Use
only the programming techniques you learned in this chapter.
// Exercise 1.30 Solution
#include <iostream>
using std::cout;
using std::endl;
using std::cin;
int main()
{
int num1, num2, num3, num4, num5, largest, smallest;
cout << "Enter five integers: ";
cin >> num1 >> num2 >> num3 >> num4 >> num5;
largest = num1;
smallest = num1;
if ( num1 > largest )
largest = num1;
if ( num2 > largest )
largest = num2;
if ( num3 > largest )
largest = num3;
if ( num4 > largest )
largest = num4;
if ( num5 > largest )
largest = num5;
if ( num1 < smallest )
smallest = num1;
if ( num2 < smallest )
smallest = num2;
if ( num3 < smallest )
smallest = num3;
if ( num4 < smallest )
smallest = num4;
if ( num5 < smallest )
smallest = num5;
cout << "Largest is " << largest
<< "\nSmallest is " << smallest << endl;
return 0;
}
Enter five integers: 88 22 8 78 21
Largest is 88
Smallest is 8
1.31 Write a program that reads an integer and determines and prints whether it is odd or even. (Hint: Use the modulus operator.
An even number is a multiple of two. Any multiple of two leaves a remainder of zero when divided by 2.)
// Exercise 1.31 Solution
#include <iostream>
using std::cout;
using std::endl;
using std::cin;
int main()
{
int num;
cout << "Enter a number: ";
cin >> num;
if ( num % 2 == 0 )
cout << "The number " << num << " is even." << endl;
if ( num % 2 != 0 )
cout << "The number " << num << " is odd." << endl;
return 0;
}
Enter a number: 73
The number 73 is odd.
1.32 Write a program that reads in two integers and determines and prints if the first is a multiple of the second. (Hint: Use the
modulus operator.)
// Exercise 1.32 Solution
#include <iostream>
using std::cout;
using std::endl;
using std::cin;
int main()
{
int num1, num2;
cout << "Enter two integers: ";
cin >> num1 >> num2;
if ( num1 % num2 == 0 )
cout << num1 << " is a multiple of " << num2 << endl;
if ( num1 % num2 != 0 )
cout << num1 << " is not a multiple of " << num2 << endl;
return 0;
}
Enter two integers: 22 8
22 is not a multiple of 8
1.33 Display a checkerboard pattern with eight output statements, then display the same pattern with as few output statements
as possible.
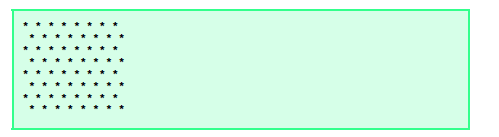
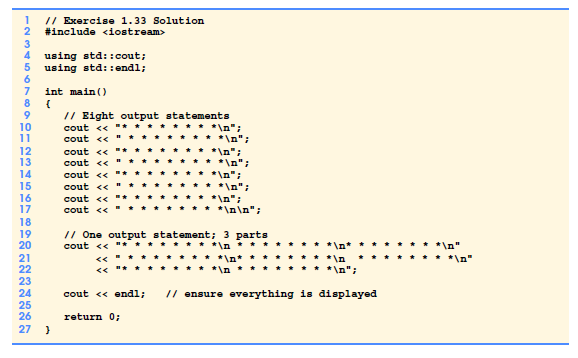
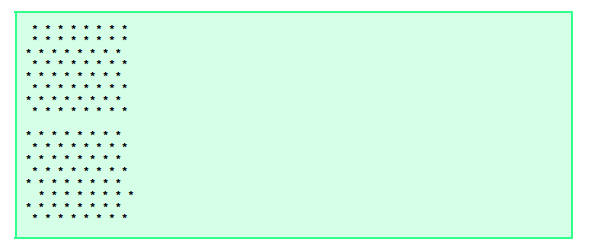
1.34 Distinguish between the terms fatal error and non–fatal error. Why might you prefer to experience a fatal error rather than
a non–fatal error?
ANS: A fatal error causes a program to terminate prematurely. A nonfatal error occurs when the logic of the program is
incorrect, and the program does not work properly. A fatal error is preferred for debugging purposes. A fatal error immediately
lets you know there is a problem with the program, whereas a nonfatal error can be subtle and possibly go undetected.
1.35 Here is a peek ahead. In this chapter you learned about integers and the type int. C++ can also represent uppercase letters,
lowercase letters and a considerable variety of special symbols. C++ uses small integers internally to represent each different character.
The set of characters a computer uses and the corresponding integer representations for those characters is called that computer’s
character set. You can print a character by simply enclosing that character in single quotes as with
cout << ‘A’; You can print the integer equivalent of a character using static_cast as follows: cout << static_cast< int >( ‘A’ );
This is called a cast operation (we formally introduce casts in Chapter 2). When the preceding statement executes, it prints the
value 65 (on systems that use the ASCII character set). Write a program that prints the integer equivalents of some uppercase letters,
lowercase letters, digits and special symbols. At a minimum, determine the integer equivalents of the following: A B C a b
c 0 1 2 $ * + / and the blank character.
// Exercise 1.35 Solution
#include <iostream>
using std::cout;
using std::endl;
using std::cin;
int main()
{
char symbol;
cout << "Enter a character: ";
cin >> symbol;
cout << symbol << "'s integer equivalent is "
<< static_cast< int > ( symbol ) << endl;
return 0;
}
Enter a character: A
A's integer equivalent is 65
1.36 Write a program that inputs a five-digit number, separates the number into its individual digits and prints the digits separated
from one another by three spaces each. (Hint: Use the integer division and modulus operators.) For example, if the user types
in 42339 the program should print
4 2 3 3 9
// Exercise 1.36 Solution
#include <iostream>
using std::cout;
using std::endl;
using std::cin;
int main()
{
int num;
cout << "Enter a five-digit number: ";
cin >> num;
cout << num / 10000 << " ";
num = num % 10000;
cout << num / 1000 << " ";
num = num % 1000;
cout << num / 100 << " ";
num = num % 100;
cout << num / 10 << " ";
num = num % 10;
cout << num << endl;
return 0;
}
Enter a five-digit number: 42339
4 2 3 3 9
1.37 Using only the techniques you learned in this chapter, write a program that calculates the squares and cubes of the numbers
from 0 to 10 and uses tabs to print the following table of values:
number square cube
0 0 0
1 1 1
2 4 8
3 9 27
4 16 64
5 25 125
6 36 216
7 49 343
8 64 512
9 81 729
10 100 1000
// Exercise 1.37 Solution
#include <iostream>
using std::cout;
using std::endl;
int main()
{
int num;
num = 0;
cout << "\nnumber\tsquare\tcube\n"
<< num << '\t' << num * num << '\t' << num * num * num << "\n";
num = num + 1;
cout << num << '\t' << num * num << '\t' << num * num * num << "\n";
num = num + 1;
cout << num << '\t' << num * num << '\t' << num * num * num << "\n";
num = num + 1;
cout << num << '\t' << num * num << '\t' << num * num * num << "\n";
num = num + 1;
cout << num << '\t' << num * num << '\t' << num * num * num << "\n";
num = num + 1;
cout << num << '\t' << num * num << '\t' << num * num * num << "\n";
num = num + 1;
cout << num << '\t' << num * num << '\t' << num * num * num << "\n";
num = num + 1;
cout << num << '\t' << num * num << '\t' << num * num * num << "\n";
num = num + 1;
cout << num << '\t' << num * num << '\t' << num * num * num << "\n";
num = num + 1;
cout << num << '\t' << num * num << '\t' << num * num * num << "\n";
num = num + 1;
cout << num << '\t' << num * num << '\t' << num * num * num << endl;
return 0;
}
1.38 Give a brief answer to each of the following “object think” questions:
a) Why does this text choose to discuss structured programming in detail before proceeding with an in-depth treatment of
object-oriented programming?
ANS: Objects are composed in part by structured program pieces.
b) What are the typical steps (mentioned in the text) of an object-oriented design process?
ANS: (1) Determine which objects are needed to implement the system. (2) Determine’s each object’s attributes. (3) Determine
each object’s behaviors. (4) Determine the interaction between the objects.
c) How is multiple inheritance exhibited by human beings?
ANS: Children. A child receives genes from both parents.
d) What kinds of messages do people send to one another?
ANS: People send messages through body language, speech, writings, email, telephones, etc.
e) Objects send messages to one another across well-defined interfaces. What interfaces does a car radio (object) present
to its user (a person object)?
ANS: Dials and buttons that allow the user to select a station, adjust the volume, adjust bass and treble, play a CD or tape,
etc.
1.39 You are probably wearing on your wrist one of the world’s most common types of objects—a watch. Discuss how each of
the following terms and concepts applies to the notion of a watch: object, attributes, behaviors, class, inheritance (consider, for example,
an alarm clock), abstraction, modeling, messages, encapsulation, interface, information hiding, data members and member
functions.
ANS: The entire watch is an object that is composed of many other objects (such as the moving parts, the band, the face, etc.) Watch attributes are time, color, band, style (digital or analog), etc. The behaviors of the watch include setting the time and getting the time. A watch can be considered a specific type of clock (as can an alarm clock). With that in mind, it is
possible that a class called Clock could exist from which other classes such as watch and alarm clock can inherit the basic features in the clock. The watch is an abstraction of the mechanics needed to keep track of the time. The user of the watch does not need to know the mechanics of the watch in order to use it; the user only needs to know that the watch keeps the
proper time. In this sense, the mechanics of the watch are encapsulated (hidden) inside the watch. The interface to the watch (its face and controls for setting the time) allows the user to set and get the time. The user is not allowed to directly touch the internal mechanics of the watch. All interaction with the internal mechanics is controlled by the interface to the watch.
The data members stored in the watch are hidden inside the watch and the member functions (looking at the face to get the time and setting the time) provide the interface to the data.
1.39 You are probably wearing on your wrist one of the world’s most common types of objects—a watch. Discuss how each of the following terms and concepts applies to the notion of a watch: object, attributes, behaviors, class, inheritance (consider, for example, an alarm clock), abstraction, modeling, messages, encapsulation, interface, information hiding, data members and member functions.
ANS: The entire watch is an object that is composed of many other objects (such as the moving parts, the band, the face, etc.) Watch attributes are time, color, band, style (digital or analog), etc. The behaviors of the watch include setting the time and getting the time. A watch can be considered a specific type of clock (as can an alarm clock). With that in mind, it is
possible that a class called Clock could exist from which other classes such as watch and alarm clock can inherit the basic features in the clock. The watch is an abstraction of the mechanics needed to keep track of the time. The user of the watch does not need to know the mechanics of the watch in order to use it; the user only needs to know that the watch keeps the
proper time. In this sense, the mechanics of the watch are encapsulated (hidden) inside the watch. The interface to the watch (its face and controls for setting the time) allows the user to set and get the time. The user is not allowed to directly touch the internal mechanics of the watch. All interaction with the internal mechanics is controlled by the interface to the watch.
The data members stored in the watch are hidden inside the watch and the member functions (looking at the face to get the time and setting the time) provide the interface to the data.
Good post. I learn something new and challenging on blogs I stumbleupon on a daily basis. Its always exciting to read content from other authors and use a little something from other web sites.
very interesting article I really like it, thanks for sharing